When you create an application, as a security measure to verify the phone number you use SMS services, or sometimes you may want to promote a discount offer where you want to send multiple messages. In order to do that you have to create a service that’ll send messages to your customers. There are a lot of services available that you can use to send SMS like Zapier, Twilio, Vonage, etc. In this tutorial, we’ll see how to send multiple Twilio SMS. But before that let us understand what Twilio is?
What is Twilio?
Twilio is a cloud communication platform as a service company that is being used by more than 200,000 users to build customer support experience using their APIs and services. It is being used by companies like Spotify, Netflix, Twitter, etc. It provides services like Twilio Flex, Twilio Frontline, Marketing Campaigns, Authy (personally I’m a big fan of this service), etc.
We have talked a lot about Twilio now. Let’s start sending multiple Twilio SMS using Python.
Prerequisites
- Python
- Twilio Account
Note: This tutorial assumes that you already have a Twilio account. If you don’t have an account you can go here and create one account.
Step -1: Getting Twilio account ID, Token, and a Phone number
Login to your Twilio account and go to console. You should see the below page:
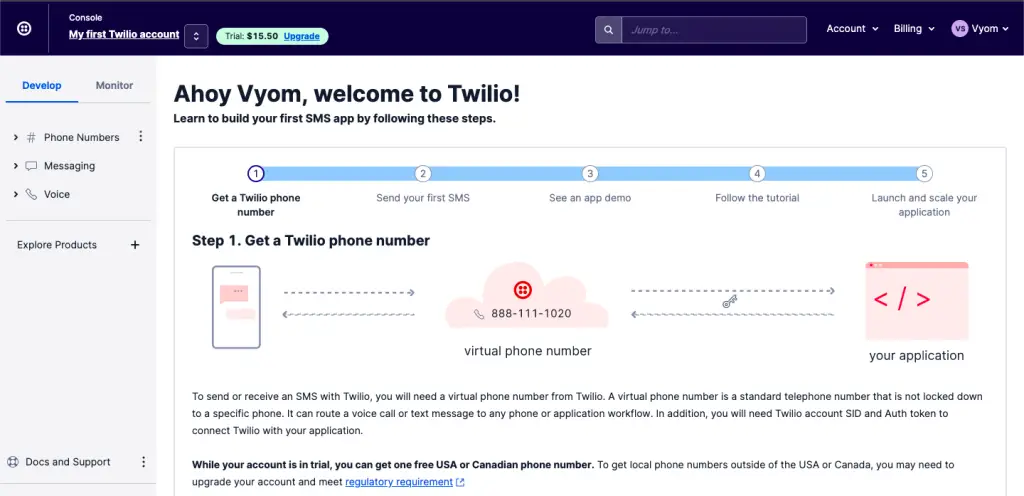
If you scroll down a bit, you should be able to see a section called “Account Info”.
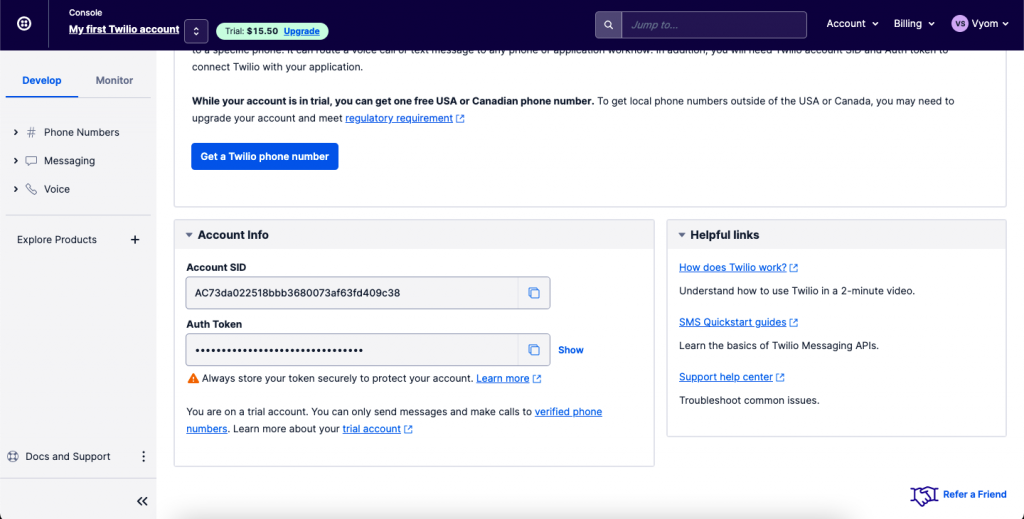
Now, copy the Account SID and Auth Token from there and paste them in a safe place (keep it handy also, because we’ll need them for this tutorial).
After that, you need to click on a button that says “Get a Twilio phone number”. It’ll generate a phone number for you. Make sure to copy the phone number also.
Step -2: Setting up the local environment
After pasting your Twilio credentials, open your Terminal (or Powershell in Windows) and create a new folder using the command:
mkdir twilio-test
cd twilio-test
Now we need to install the official SDK that comes with Twilio. It makes things a lot easier when it comes to sending Twilio SMS or using any other service from Twilio.
Use the below command to install the SDK:
python3 -m pip install twilio
After installing SDK, create a file called sendsms.py
and open it in VS Code.
Step -3: Writing the code to send single Twilio SMS
In the file sendsms.py
paste the below code:
# Download the helper library from https://www.twilio.com/docs/python/install
import os
from twilio.rest import Client
# Find your Account SID and Auth Token at twilio.com/console
# and set the environment variables. See http://twil.io/secure
account_sid = YOUR_ACCOUNT_SID_HERE
auth_token = YOUR_AUTH_TOKEN_HERE
client = Client(account_sid, auth_token)
phone_number = ‘RECIEVER_PHONE_NUMBER’
message = client.messages \
.create(
body="Join Earth's mightiest heroes. Like Kevin Bacon.",
from_='TWILIO_GENERATED_PHONE_NUMBER’,
to=phone_number
)
print(message.sid)
You can find the same code here also.
Note: You need to replace RECIEVER_PHONE_NUMBER
, TWILIO_GENERATED_PHONE_NUMBER
, YOUR_ACCOUNT_SID_HERE
, and YOUR_AUTH_TOKEN_HERE
with their respective values.
Code Explanation
We’re first importing all the dependencies and then we’re creating the Twilio client. After that, we’re calling the function called messages()
that takes the body of the message, the sender’s number, and the receiver’s number to send the SMS.
Output
To execute the code, use the command:
python3 sendsms.py
On successful execution, it should print a unique id like the below screenshot:

Step -4: Writing the code to send multiple Twilio SMS
Now open the same file i.e. `sendsms.py` in VS Code and replace the existing code with the below code:
import os
from twilio.rest import Client
# Find your Account SID and Auth Token at twilio.com/console
# and set the environment variables. See http://twil.io/secure
account_sid = YOUR_ACCOUNT_SID_HERE
auth_token = YOUR_AUTH_TOKEN_HERE
client = Client(account_sid, auth_token)
phone_numbers = ['PHONE_NUMBER_1’, ‘PHONE_NUMBER_2’']
for phone_number in phone_numbers:
message = client.messages \
.create(
body="Join Earth's mightiest heroes. Like Kevin Bacon.",
from_='TWILIO_GENERATED_PHONE_NUMBER’,
to=phone_number
)
print(message.sid)
Note: You need to replace TWILIO_GENERATED_PHONE_NUMBER
, YOUR_ACCOUNT_SID_HERE
, and YOUR_AUTH_TOKEN_HERE
with their respective values. Since the variable phone_numbers
is a list now, so you need to pass multiple phone numbers as a list of strings.
Output
To execute the code, use the command:
python3 sendsms.py
On successful execution, it should print multiple unique ids like the below screenshot:

Final Words
Sending multiple SMS using Twilio is a lot easier. Their SDK and documentation make things much easier. A lot of companies rely on Twilio for these functionalities as they’re very reliable and affordable. You can even send WhatsApp and Facebook messages using Twilio.
Here are some useful tutorials that you can read:
- Concurrency in Python
- Basic Neural Network in Python to Make Predictions
- Monitor Python scripts using Prometheus
- Test Your Typing Speed Using Python
- Instagram Hashtag Generator in Python
- How to create a Word Guessing Game in Python
- Convert an image to 8-bit image
- Schedule Python Scripts with Apache Airflow
- Sudoku game in Python using Pygame
- How to Deploy Flask API on Heroku?
- How to Update your Mac Address using Python